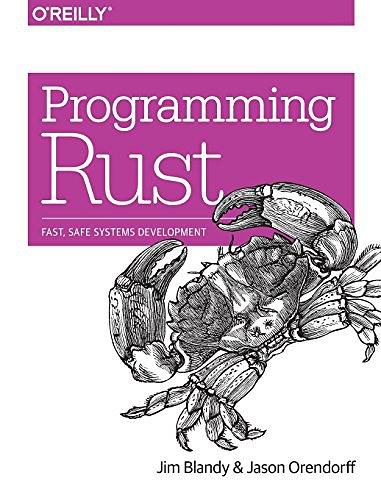
章节目录
Preface
1. Why Rust?
Type Safety
2. A Tour of Rust
Downloading and Installing Rust
A Simple Function
Writing and Running Unit Tests
Handling Command-Line Arguments
A Simple Web Server
Concurrency
3. Basic Types
Machine Types
Tuples
Pointer Types
Arrays, Vectors, and Slices
String Types
Beyond the Basics
4. Ownership
Ownership
Moves
Copy Types: The Exception to Moves
Rc and Arc: Shared Ownership
5. References
References as Values
Reference Safety
Sharing Versus Mutation
Taking Arms Against a Sea of Objects
6. Expressions
An Expression Language
Blocks and Semicolons
Declarations
if and match
Loops
return Expressions
Why Rust Has loop
Function and Method Calls
Fields and Elements
Reference Operators
Arithmetic, Bitwise, Comparison, and Logical Operators
Assignment
Type Casts
Closures
Precedence and Associativity
Onward
7. Error Handling
Panic
Result
8. Crates and Modules
Crates
Modules
Turning a Program into a Library
The src/bin Directory
Attributes
Tests and Documentation
Specifying Dependencies
Publishing Crates to crates.io
Workspaces
More Nice Things
9. Structs
Named-Field Structs
Tuple-Like Structs
Unit-Like Structs
Struct Layout
Defining Methods with impl
Generic Structs
Structs with Lifetime Parameters
Deriving Common Traits for Struct Types
Interior Mutability
10. Enums and Patterns
Enums
Patterns
The Big Picture
11. Traits and Generics
Using Traits
Defining and Implementing Traits
Fully Qualified Method Calls
Traits That Define Relationships Between Types
Reverse-Engineering Bounds
Conclusion
12. Operator Overloading
Arithmetic and Bitwise Operators
Equality Tests
Ordered Comparisons
Index and IndexMut
Other Operators
13. Utility Traits
Drop
Sized
Clone
Copy
Deref and DerefMut
Default
AsRef and AsMut
Borrow and BorrowMut
From and Into
ToOwned
Borrow and ToOwned at Work: The Humble Cow
14. Closures
Capturing Variables
Function and Closure Types
Closure Performance
Closures and Safety
Callbacks
Using Closures Effectively
15. Iterators
The Iterator and IntoIterator Traits
Creating Iterators
Iterator Adapters
Consuming Iterators
Implementing Your Own Iterators
16. Collections
Overview
Vec<T>
VecDeque<T>
LinkedList<T>
BinaryHeap<T>
HashMap<K, V> and BTreeMap<K, V>
HashSet<T> and BTreeSet<T>
Hashing
Beyond the Standard Collections
17. Strings and Text
Some Unicode Background
Characters (char)
String and str
Formatting Values
Regular Expressions
Normalization
18. Input and Output
Readers and Writers
Files and Directories
Networking
19. Concurrency
Fork-Join Parallelism
Channels
Shared Mutable State
What Hacking Concurrent Code in Rust Is Like
20. Macros
Macro Basics
Built-In Macros
Debugging Macros
The json! Macro
Avoiding Syntax Errors During Matching
Beyond macro_rules!
21. Unsafe Code
Unsafe from What?
Unsafe Blocks
Unsafe Functions
Unsafe Block or Unsafe Function?
Undefined Behavior
Unsafe Traits
Raw Pointers
Foreign Functions: Calling C and C++ from Rust
Conclusion
Index
内容简介
This practical book introduces systems programmers to Rust, the new and cutting-edge language that’s still in the experimental/lab stage. You’ll learn how Rust offers the rare and valuable combination of statically verified memory safety and low-level control—imagine C++, but without dangling pointers, null pointer dereferences, leaks, or buffer overruns.
Author Jim Blandy—the maintainer of GNU Emacs and GNU Guile—demonstrates how Rust has the potential to be the first usable programming language that brings the benefits of an expressive modern type system to systems programming. Rust’s rules for borrowing, mutability, ownership, and moves versus copies will be unfamiliar to most systems programmers, but they’re key to Rust’s unique advantages.
This book presents Rust’s rules clearly and economically; elaborates on their consequences; and shows you how to express the programs you want to write in terms that Rust can prove are free of a broad class of common errors.
下载说明
1、Programming Rust是作者Jim Blandy / Jason Orendorff创作的原创作品,下载链接均为网友上传的网盘链接!
2、相识电子书提供优质免费的txt、pdf等下载链接,所有电子书均为完整版!
下载链接
热门评论
-
逆铭睡眼惺忪地的评论当枕边书读完了,除了中间有些地方介绍API比较枯燥,对核心概念的讲解、对不同做法的利弊分析都非常清晰,娓娓道来的风格读起来也很舒服
-
DigitalTree的评论很棒的书,主要面向有经验的程序员(特别是 C++ 程序员),有些地方没有官方的书讲得详细。其实最后一章 Unsafe Code 没看完,以后再看。。。600页不到的书为了公司培训做了200多页PPT,熬了不少夜,也是醉了。。。
-
龙三的评论比官方 doc 好
-
HariSeldon的评论比官方好
-
春你麻p晚的评论是、、、恋爱的感觉
-
paranoid.emacs的评论我觉得最好的系统语言还是modern c++.
-
xiaq的评论讲 trait 的地方能让人感觉到 rust 是一个还没设计完的语言。
-
的评论写的真一般,而且 trait 整个地方都变了...
-
10/10=1的评论读到了对Rust更详细的认识。
-
lambeta的评论Rust 是一门系统编程语言,也是许多区块链底层编程语言,不论是旧欢 Parity,还是新贵 Libra;不论是微软还是 Linux 的核心开发者都对它青眼有加。 Rust 有一些很迷人的地方,比如:AOT,内存安全性,空指针安全性,还是丰富的类型系统,异或是庞大的社区生态。这些别的语言也有,但是都没有这么彻底。 Rust 的特点也十分突出,Ownership 和 borrow、references 还有 shadow,令人眼花缭乱,最最有趣的就是你写一段程序,编译器会 blame 你很多,你就感觉是在和编译器搏斗。 学习 Rust 不只是一时兴起(早在2015年我就听闻过),也是一种拥抱变化的态度,重要的是可以让你在看众多区块链代码时不会那么心慌。 语言的趋势反映了未来的主流开发群体
-
阿丹的评论循序渐进,入门不错。
-
cassc的评论第二本rust读物。 和官方那本一起读勉强rust入门
-
qwertydvorak的评论细节很多,不如官网那本《the rust programming language》易读。有很多跟 C++ 的对比。本书使用 rustc 1.17.0, 小部分内容已经变成 deprecated, 比如现在 trait object 要求明确使用 dyn 关键字
-
beautifularea的评论Programming Rust
-
AperSpike的评论读过官网"The book"之后再来看的这本书,内容深度和广度都要比"The book"好
-
can.的评论比官方那本好,虽然看完还是觉得C++好复杂
-
ˊ﹎If i…°的评论与C++对比着进行讲解,很赞
-
E-Neo的评论以后自己的项目需要 C/C++ 的时候就用 Rust 了。
-
Ruby的评论醍醐灌顶!见过的最好的rust教程(甚于官方文档)
-
FXTi的评论其实看的是Rust book的2019.5.17的nightly版本,但是没有出版
热门推荐
- 1 独腿旅行者
- 2 人间正道
- 3 野兽必死
- 4 森林中有许多酒
- 5 鲁迅传
- 6 动物园长夫人
- 7 不再努力成为另一个人
- 8 热爱生活的一万个理由
- 9 专家的窘境
- 10 艺术的旅程